Validation allows you to define certain rules for your fields. For example, you can specify that a field is required so if the user does not complete the field, an error is displayed and the user cannot add the product to the cart.
By default, fields are validated when the user clicks the Add to Cart button. WooCommerce’s standard behaviour is to validate the add to cart form and reload the product page. Any validation notices are displayed when the page is reloaded.
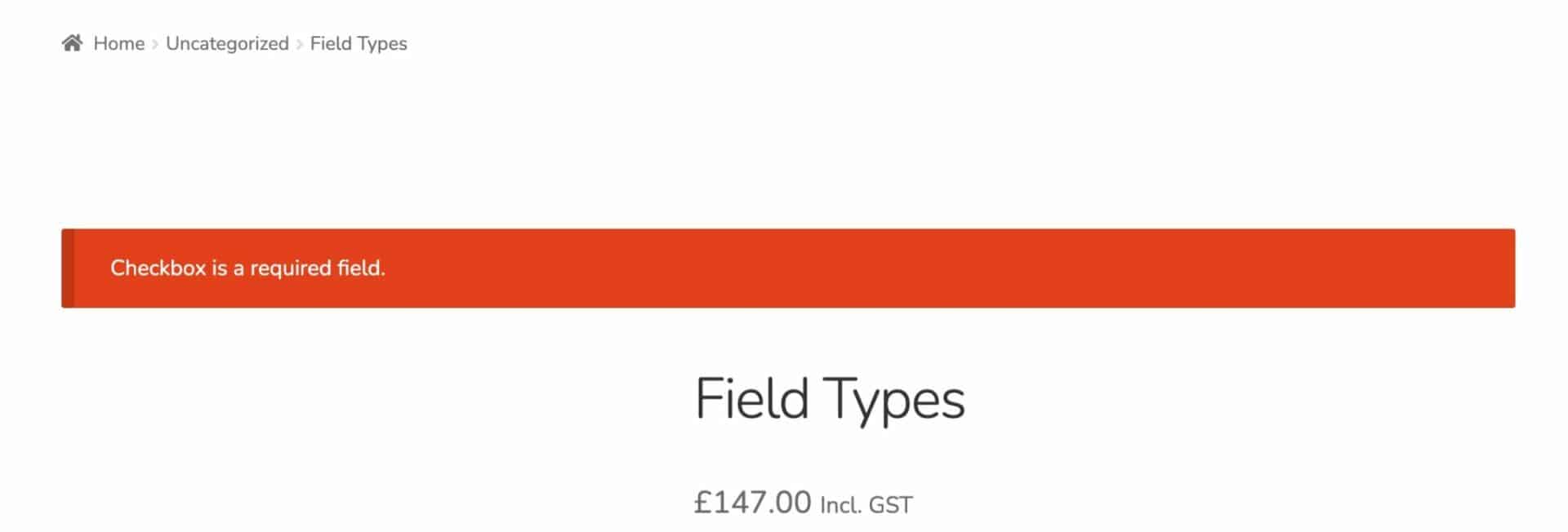
The position and style of the notice is set by your theme.
Optimised validation
If you prefer the validation to take place without the product reloading, you can choose the ‘Optimised validation’ option. Go to WooCommerce > Settings > Product Add-Ons to enable the setting.

Optimised validation will display inline messages for any fields that have failed validation when the user clicks the Add to Cart button.
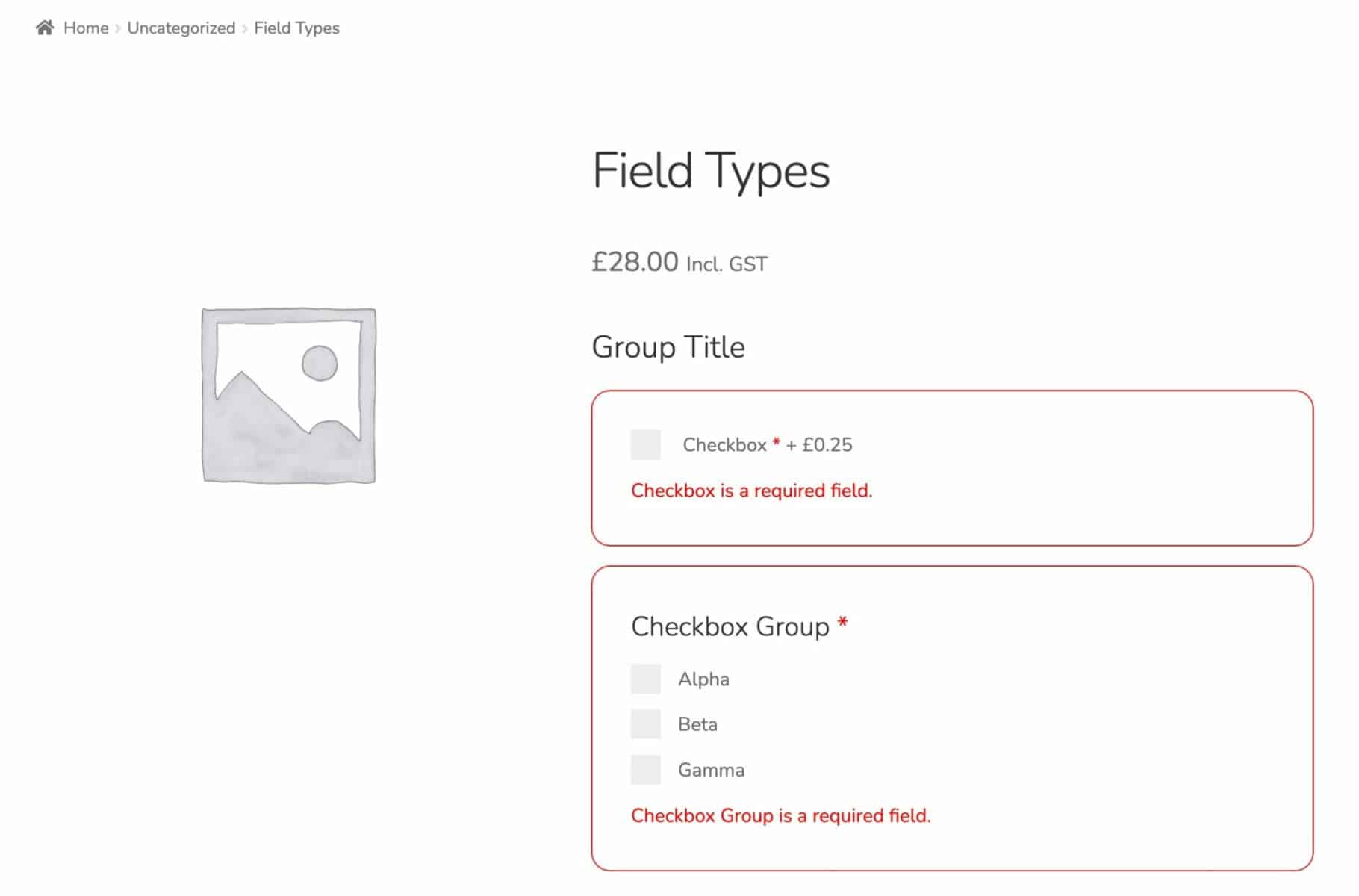
This helps guide the user to any fields which have failed validation. It’s particularly helpful on product pages with several add-on fields.
Filter optimised validation messages
If you’d like to change the text in the optimised validation messages, you can use these snippets:
<?php | |
/** | |
* Filter exact children validation notice | |
*/ | |
function prefix_exact_children_validation_notice( $notice, $label, $min_products ) { | |
$notice = sprintf( | |
__( '%s requires you to choose %s products', 'pewc' ), | |
esc_html( $label ), | |
$min_products | |
); | |
return $notice; | |
} | |
add_action( 'pewc_filter_exact_children_validation_notice', 'prefix_exact_children_validation_notice', 10, 3 ); | |
/** | |
* Filter min children validation notice | |
*/ | |
function prefix_min_children_validation_notice( $notice, $label, $min_products ) { | |
$notice = sprintf( | |
__( '%s requires you to choose %s products', 'pewc' ), | |
esc_html( $label ), | |
$min_products | |
); | |
return $notice; | |
} | |
add_action( 'pewc_filter_min_children_validation_notice', 'prefix_min_children_validation_notice', 10, 3 ); | |
/** | |
* Filter max children validation notice | |
*/ | |
function prefix_max_children_validation_notice( $notice, $label, $min_products ) { | |
$notice = sprintf( | |
__( '%s requires you to choose %s products', 'pewc' ), | |
esc_html( $label ), | |
$min_products | |
); | |
return $notice; | |
} | |
add_action( 'pewc_filter_max_children_validation_notice', 'prefix_max_children_validation_notice', 10, 3 ); |
What happens if validation isn’t working?
If the user is able to add a product to the cart and bypass validation, either:
- Enable ‘Optimised validation’ above. This will prevent the user from adding the product to the cart
- Or, check this article
Number field validation
If you have set a minimum or maximum value for your number field, it will try to validate this whether or not the field is required.
This means that if you have entered a minimum of 5 and the user leaves the field empty, there will still be a validation error if the user tries to add the product to the cart – even though the field is not required.
To ensure that number fields can be left empty even if they have a minimum or maximum value, and prevent validation on a non-required number field, add this to your functions.php file or snippets file:
<?php | |
// Add this if you want to prevent non-required number fields from validating min or max values | |
add_filter( 'pewc_only_validate_number_field_value_if_field_required', '__return_true' ); | |
?> |
Filter validation message
You can filter the default validation error message when the user adds an item to the cart like this:
<?php | |
/** | |
* @param $message The validation message | |
* @param $label The field label | |
* @param $item The field object | |
*/ | |
function prefix_filter_validation_notice( $message, $label, $item ) { | |
$message = __( 'My new validation message for' ); | |
$message .= $label; | |
return $message; | |
} | |
add_filter( 'pewc_filter_validation_notice', 'prefix_filter_validation_notice', 10, 3 ); |
Custom validation for add-on fields
You can add your own validation when a user adds a product to their cart.
Validation minimum number of files
In the example below, we’re checking that the value of field with ID pewc_group_3990_4033 is not less than 10. Substitute with your own values.
<?php | |
/** | |
* Custom validation for add-on fields | |
*/ | |
function prefix_filter_validate_cart_item_status( $passed, $post, $item ) { | |
// pewc_group_3990_4033 is the ID of the field | |
if( isset( $post['pewc_group_3990_4033'] ) && floatval( $post['pewc_group_3990_4033'] ) < 10 ) { | |
wc_add_notice( 'My validation message', 'error' ); | |
$passed = false; | |
} | |
return $passed; | |
} | |
add_filter( 'pewc_filter_validate_cart_item_status', 'prefix_filter_validate_cart_item_status', 10, 3 ); |
Here’s an example for setting a minimum number of files to upload:
<?php | |
/** | |
* Custom validation to set minimum number of uploads | |
*/ | |
function prefix_check_minimum_uploads( $passed, $post, $item ) { | |
// Enter the Group ID for the upload field | |
$group_id = 2908; | |
// Enter the Field ID of the upload field | |
$field_id = 2909; | |
// Set the minimum number of uploads required | |
$min_uploads = 3; | |
// Set your message if the user doesn't upload enough files | |
$message = 'Please upload 6 files'; | |
$field_name = 'pewc_group_' . $group_id . '_' . $field_id . '_number_uploads'; | |
if( isset( $post[$field_name] ) && floatval( $post[$field_name] ) < $min_uploads ) { | |
wc_add_notice( $message, 'error' ); | |
$passed = false; | |
} | |
return $passed; | |
} | |
add_filter( 'pewc_filter_validate_cart_item_status', 'prefix_check_minimum_uploads', 10, 3 ); |
Setting minimum quantity of child products
Here’s an example for requiring a minimum quantity of child products:
<?php | |
/** | |
* Custom validation for add-on fields | |
*/ | |
function prefix_validate_total_child_items( $passed, $product_id, $quantity, $variation_id=null, $cart_item_data=array() ) { | |
// The parent product ID that we need to validate | |
$parent_product_ID = 9059; // Change this | |
if( $parent_product_ID != $product_id ) { | |
return $passed; | |
} | |
// The list of field IDs that need to be checked | |
$fields = array( 9061 ); // Change this | |
// The combined quantity | |
$total = 0; | |
// The required quantity | |
$required = 15; // Change this | |
// Iterate through each field and get combined quantity | |
$groups = pewc_get_extra_fields( $product_id ); | |
if( $groups ) { | |
foreach( $groups as $group_id=>$group ) { | |
if( isset( $group['items'] ) ) { | |
foreach( $group['items'] as $field_id=>$field ) { | |
$id = $field['id']; | |
if( in_array( $field_id, $fields ) ) { | |
// This is one of our fields | |
$child_products = ! empty( $field['child_products'] ) ? $field['child_products'] : array(); | |
foreach( $child_products as $key=>$child_product_id ) { | |
if( isset( $_POST[$id . '_child_quantity_' . $child_product_id] ) ) { | |
$total += $_POST[$id . '_child_quantity_' . $child_product_id]; | |
} | |
} | |
} | |
} | |
} | |
} | |
} | |
if( $total < $required ) { | |
wc_add_notice( 'My validation message', 'error' ); // Change this | |
$passed = false; | |
} | |
return $passed; | |
} | |
add_filter( 'woocommerce_add_to_cart_validation', 'prefix_validate_total_child_items', 10, 5 ); |
Setting maximum quantity of child products
Here’s an example of how to filter the validation message when the maximum number of child products has been exceeded.
<?php | |
/** | |
* Filter validation message for maximum number of child products | |
*/ | |
function prefix_filter_max_children_validation_notice( $message, $field_label, $max_products ) { | |
// You can write a custom message here | |
// $field_label is the name of the field | |
// $max_products is the maximum number of products allowed | |
return $message; | |
} | |
add_filter( 'pewc_filter_max_children_validation_notice', 'prefix_filter_max_children_validation_notice', 10, 3 ); |
You can update the $message variable
to whatever you like. Use the $field_label
and $max_products
variables in your message if required.
Find out here how to add this snippet to your site.